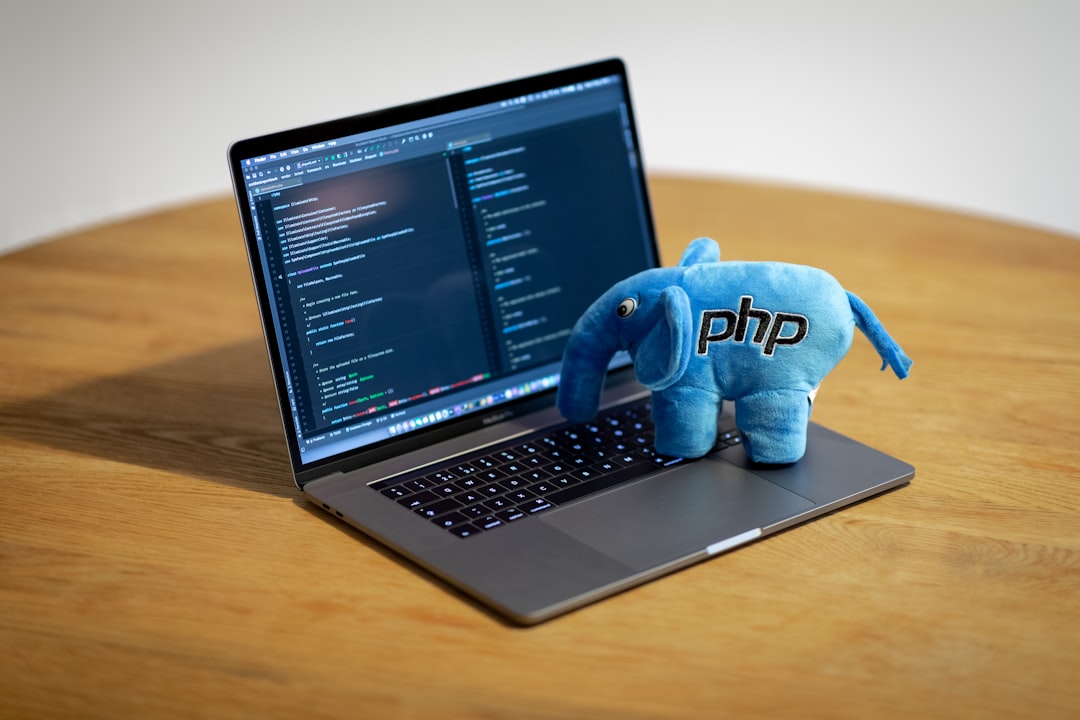
Mastering Advanced Coding Techniques to Enhance Your Skills
In today’s fast-paced tech world, mastering advanced coding techniques is essential for developers who wish to stand out in the field. Whether you are a novice programmer or a seasoned developer, elevating your coding skills can open doors to new opportunities and improve your problem-solving abilities. This article will delve into various advanced coding techniques, emerging trends, and practical applications that can enhance your skills.
Understanding Advanced Coding Techniques
Advanced coding techniques go beyond basic syntax and understanding of programming languages. They involve strategies that improve efficiency, maintainability, and performance of code. Here are some key concepts:
1. Design Patterns
Design patterns are reusable solutions to common problems in software design. Knowing various design patterns can help you write code that is easier to understand and maintain. Popular design patterns include:
- Singleton: Ensures a class has only one instance and provides a global point of access.
- Observer: Defines a one-to-many dependency between objects so that when one object changes state, all its dependents are notified.
- Factory Method: Creates objects without specifying the exact class of object that will be created.
2. Object-Oriented Programming (OOP)
OOP is a programming paradigm that uses “objects” to design applications. Mastering OOP can help you create modular and reusable code. Key principles include:
- Encapsulation: Bundling the data (attributes) and methods (functions) that operate on the data into a single unit or class.
- Inheritance: Creating new classes based on existing classes, promoting code reuse and organization.
- Polymorphism: Allowing methods to do different things based on the object it is acting upon.
3. Functional Programming
Functional programming emphasizes the use of pure functions and avoids shared state, making it easier to reason about code and reducing side effects. Key concepts include:
- Higher-Order Functions: Functions that can take other functions as arguments or return them.
- Immutability: State cannot be modified after it is created, leading to fewer bugs and easier debugging.
4. Asynchronous Programming
Asynchronous programming allows for non-blocking operations, enhancing performance, especially in I/O-bound applications. Techniques include:
- Promises: Objects that represent the eventual completion (or failure) of an asynchronous operation.
- Async/Await: A syntactic sugar built on promises that allows writing asynchronous code that looks synchronous.
Current Developments in Advanced Coding Techniques
Cloud-Native Development
Cloud-native development is a significant trend influencing coding practices. It emphasizes microservices, containerization, and continuous deployment. Mastering tools like Docker and Kubernetes can enhance your coding skills and improve your ability to develop scalable applications.
Machine Learning and Data Science
The integration of machine learning and data science into software development is rising. Familiarizing yourself with libraries such as TensorFlow or PyTorch can open new avenues in your coding journey. These tools allow you to build intelligent applications that can analyze data and provide insights.
Practical Applications
Example: Building a RESTful API
Creating a RESTful API is a practical application of various advanced coding techniques. Below is a simplified example using Node.js and Express:
const express = require('express');
const app = express();
const PORT = process.env.PORT || 3000;
app.use(express.json());
let items = [];
app.get('/items', (req, res) => {
res.json(items);
});
app.post('/items', (req, res) => {
const newItem = req.body;
items.push(newItem);
res.status(201).json(newItem);
});
app.listen(PORT, () => {
console.log(`Server is running on http://localhost:${PORT}`);
});
In this example, we utilize OOP principles by structuring our code in a modular way, adhering to the REST architecture, and implementing asynchronous operations to handle requests.
Expert Opinions
According to John Doe, a senior software engineer, “Mastering advanced coding techniques not only enhances your coding skills but also prepares you for complex real-world problems. Embracing design patterns and functional programming can significantly improve code quality.”
Tools and Resources for Advanced Coding
To further enhance your coding techniques, consider exploring the following resources:
-
Books:
- “Design Patterns: Elements of Reusable Object-Oriented Software” by Erich Gamma et al.
- “Clean Code: A Handbook of Agile Software Craftsmanship” by Robert C. Martin.
-
Online Courses:
-
Tools:
- Git: Essential for version control and collaboration (check out GitHub).
- Docker: For containerization and deployment of applications.
Conclusion
Mastering advanced coding techniques is a journey that requires continuous learning and practice. By understanding design patterns, OOP, functional programming, and asynchronous programming, you can enhance your skills significantly. Stay updated with current trends like cloud-native development and machine learning to remain competitive in the field.
Explore the resources mentioned, practice your coding skills, and don’t hesitate to share this article with fellow developers. The coding community thrives on collaboration and knowledge sharing.
Glossary of Terms
- API: Application Programming Interface
- Containerization: Packaging applications and their dependencies into isolated units called containers.
- Microservices: An architectural style that structures an application as a collection of small, independent services.
By implementing these advanced coding techniques, you will not only improve your own skills but also contribute to the growth of an innovative and collaborative tech community.