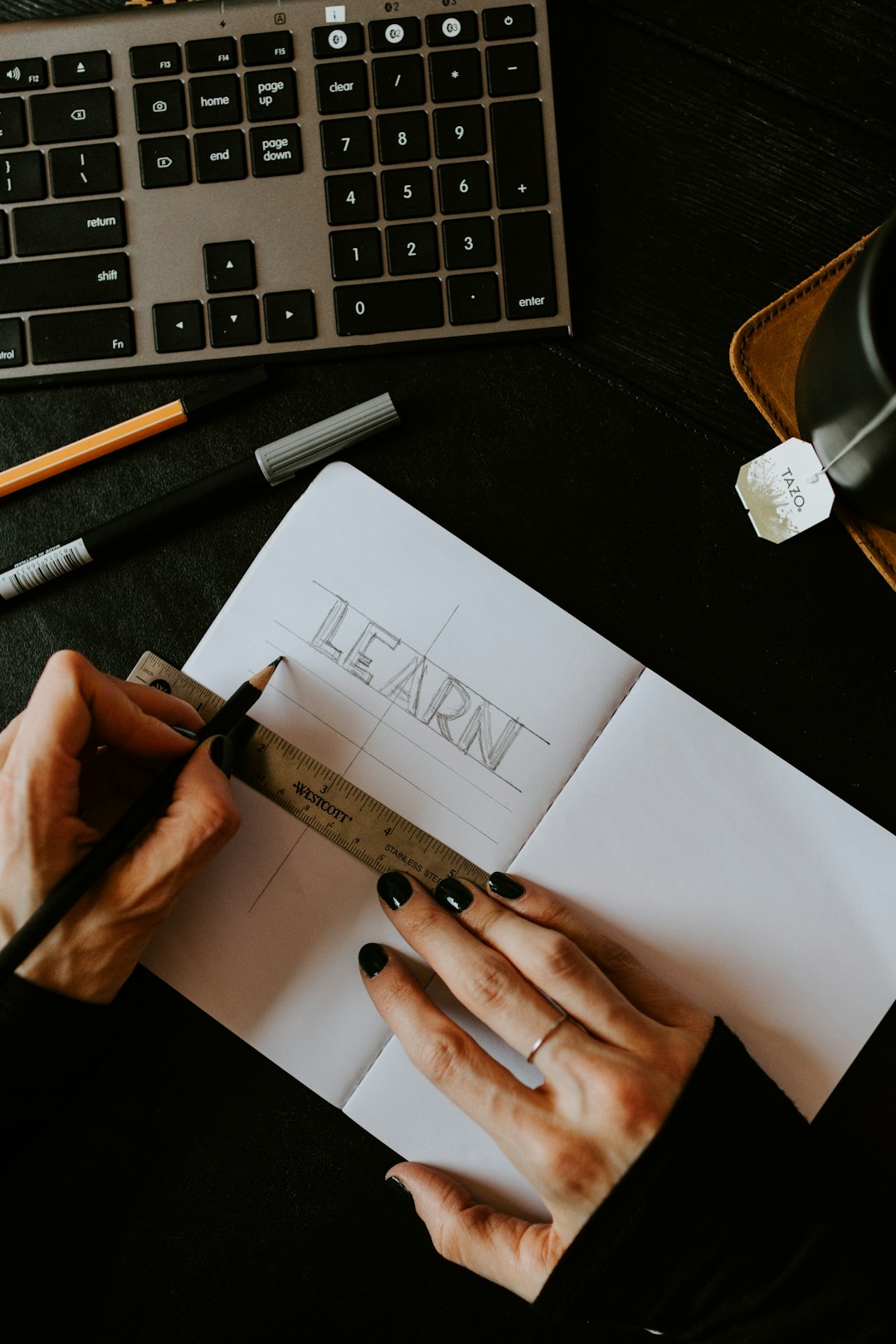
Mastering Coding Fundamentals for Beginners: A Comprehensive Guide
Coding has become an essential skill in today’s digital world, transcending industries and creating numerous opportunities. For beginners, mastering coding fundamentals lays the foundation for a successful programming journey. This guide delves into the essential concepts, resources, and practices that every aspiring coder should know.
Understanding the Basics of Coding
What is Coding?
Coding, or programming, involves writing instructions for a computer to execute. These instructions are written in a specific programming language, which can be understood by computers. Popular languages for beginners include Python, JavaScript, and Ruby.
Why Learning Coding Fundamentals is Crucial
Mastering coding fundamentals equips you with the skills to solve problems, think logically, and create applications. It helps in developing analytical thinking and improves your ability to communicate complex ideas clearly.
Key Concepts Every Beginner Should Know
1. Variables and Data Types
Variables are used to store data that can be changed during program execution. Understanding different data types like integers, strings, and booleans is vital.
# Example in Python
age = 25 # Integer
name = "Alice" # String
is_student = True # Boolean
2. Control Structures
Control structures like loops and conditionals allow you to dictate the flow of a program. Mastering these concepts will enable you to write more complex code.
# Example of a conditional statement in Python
if age >= 18:
print("You are an adult.")
else:
print("You are a minor.")
3. Functions
Functions are reusable blocks of code that perform a specific task. They help in organizing code and making it more manageable.
# Example of a function in Python
def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
4. Data Structures
Understanding data structures such as lists, dictionaries, and sets is crucial for efficient data handling.
# Example of a list in Python
fruits = ["apple", "banana", "cherry"]
5. Debugging and Testing
Learning to debug and test your code is essential. Debugging tools and practices help identify and fix errors, ensuring your code runs smoothly.
Practical Applications of Coding Fundamentals
Building Simple Projects
One of the best ways to reinforce your coding fundamentals is by building small projects. Start with simple applications like a calculator or a to-do list app. These projects allow you to apply what you’ve learned practically.
Collaborating on Open Source
Engaging in open-source projects on platforms like GitHub enhances your coding skills and allows you to learn from experienced developers. Contributing to projects can also expand your professional network.
Emerging Trends in Coding Education
As technology evolves, so does the approach to learning coding. Online platforms and coding bootcamps have gained popularity, offering interactive lessons tailored to beginners. Resources such as freeCodeCamp, Codecademy, and Coursera provide beginner-friendly courses that cover essential coding fundamentals.
Expert Opinions
According to industry experts, a strong grasp of coding fundamentals is essential for any aspiring developer. “Understanding the core concepts of programming will make it easier to learn new languages and technologies in the future,” says Jane Doe, a leading software engineer.
Resources for Further Learning
To expand your knowledge, consider these tools and resources:
- freeCodeCamp: Offers free coding tutorials.
- Codecademy: Interactive coding lessons for various programming languages.
- Coursera: Online courses from top universities.
- Khan Academy: Free resources for learning programming basics.
Glossary of Terms
- Variable: A storage location identified by a name that can hold data.
- Function: A reusable piece of code that performs a specific task.
- Loop: A control structure that repeats a block of code multiple times.
Mastering coding fundamentals is a journey that requires patience and practice. By focusing on these core concepts, utilizing available resources, and engaging in hands-on projects, beginners can build a strong foundation in programming. As you progress, don’t hesitate to share your knowledge with others and seek guidance when needed.
If you found this guide helpful, consider subscribing to our newsletter for more insights into coding and technology trends. Happy coding!