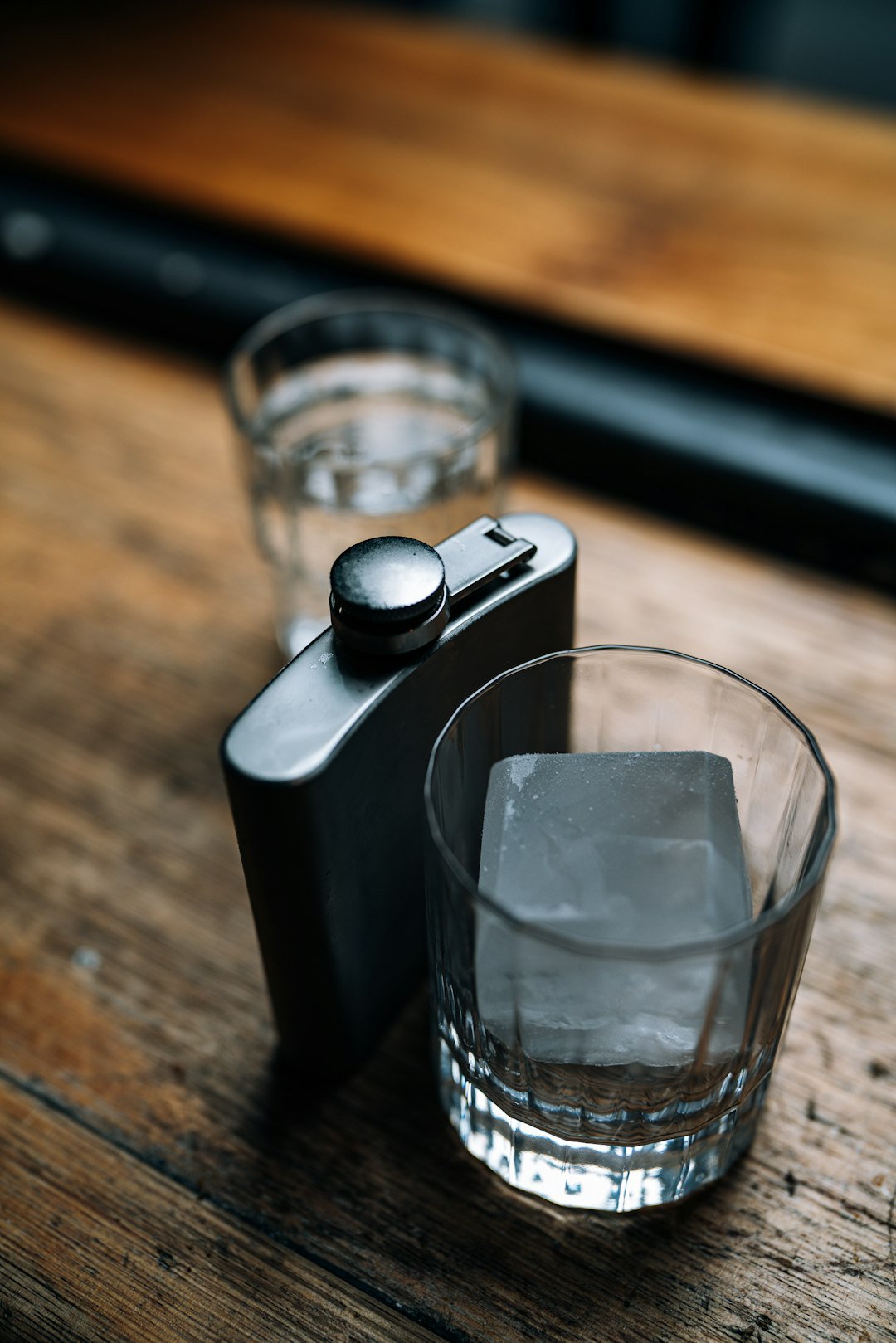
Optimize Your Flask API with Efficient Routing Using SQLAlchemy Models
In today’s fast-paced development environment, optimizing your Flask API is crucial for performance and scalability. Leveraging SQLAlchemy models for efficient routing can significantly enhance your API’s responsiveness and maintainability. This article explores how to optimize your Flask API routing while utilizing SQLAlchemy models effectively.
Understanding Flask and SQLAlchemy
Flask is a lightweight WSGI web application framework in Python. It’s designed for simplicity and flexibility, making it a popular choice for developing APIs. SQLAlchemy, on the other hand, is an ORM (Object Relational Mapper) that facilitates database interactions in Python applications.
Combining Flask with SQLAlchemy allows developers to create data-driven applications with less boilerplate code, enabling easier maintenance and rapid development. However, to optimize your API performance, you must consider how you structure your routes and manage your data queries.
Efficient Routing in Flask
Routing is a critical aspect of any web application. It dictates how requests are handled and how responses are generated. In Flask, routes are defined using decorators. To optimize routing, consider the following strategies:
1. Use Flask Blueprints
Flask Blueprints allow you to organize your routes into manageable sections. This modular approach makes it easier to maintain and scale your application. For example:
from flask import Blueprint
api = Blueprint('api', __name__)
@api.route('/users', methods=['GET'])
def get_users():
# Logic to retrieve users
pass
This method not only organizes your code but also improves the loading times of your application since only necessary routes are registered when required.
2. Consolidate Routes
Avoid duplicating routes that serve similar purposes. Instead, use query parameters to handle variations in requests. For instance, instead of having separate routes for fetching active and inactive users, you could have a single route like this:
@api.route('/users', methods=['GET'])
def get_users():
status = request.args.get('status', default='active') # 'active' or 'inactive'
# Logic to retrieve users based on status
pass
3. Optimize Query Performance with SQLAlchemy
SQLAlchemy models provide a robust way to interact with your database. However, poorly constructed queries can lead to performance bottlenecks. Here are some best practices:
a. Use Eager Loading
Eager loading allows you to load related data in a single query, minimizing the number of database hits. This is particularly useful in scenarios where you need to access related entities. For example, if you have a User
model with a related Post
model:
users = session.query(User).options(joinedload(User.posts)).all()
This query retrieves users along with their posts in a single database call.
b. Limit the Data Returned
When querying data, always return only the fields necessary for your application. This reduces the amount of data transferred and speeds up response times:
users = session.query(User.id, User.username).all()
4. Implement Caching
Caching can dramatically improve the performance of your API by storing frequently accessed data. Flask-Caching is an extension that integrates seamlessly with Flask applications. You can use it like this:
from flask_caching import Cache
cache = Cache(app)
@cache.cached(timeout=60)
@api.route('/users', methods=['GET'])
def get_users():
# Logic to retrieve users
pass
This example caches the results for 60 seconds, reducing the load on your database for repeated requests.
Emerging Trends in Flask API Development
As the demand for efficient APIs grows, several trends are emerging in the Flask ecosystem:
- Microservices Architecture: More developers are adopting microservices, where APIs are designed as independent services. This allows for better scalability and fault isolation.
- GraphQL Integration: With the rise of GraphQL, developers are looking for ways to integrate it with Flask to provide more flexible API responses.
- Asynchronous Programming: Leveraging async features in Flask can lead to better performance, especially in I/O-bound applications.
Further Reading and Resources
To expand your knowledge on optimizing Flask APIs with SQLAlchemy, consider the following resources:
These resources provide in-depth information on best practices and advanced techniques.
Conclusion
Optimizing your Flask API with efficient routing and SQLAlchemy models is essential for developing high-performance applications. By implementing best practices like using Blueprints, consolidating routes, optimizing queries, and leveraging caching, you can significantly improve your API’s responsiveness and ease of maintenance.
Stay updated with emerging trends and continuously refine your approach to meet the evolving needs of your application. Don’t forget to share this article with your peers who might benefit from these insights!