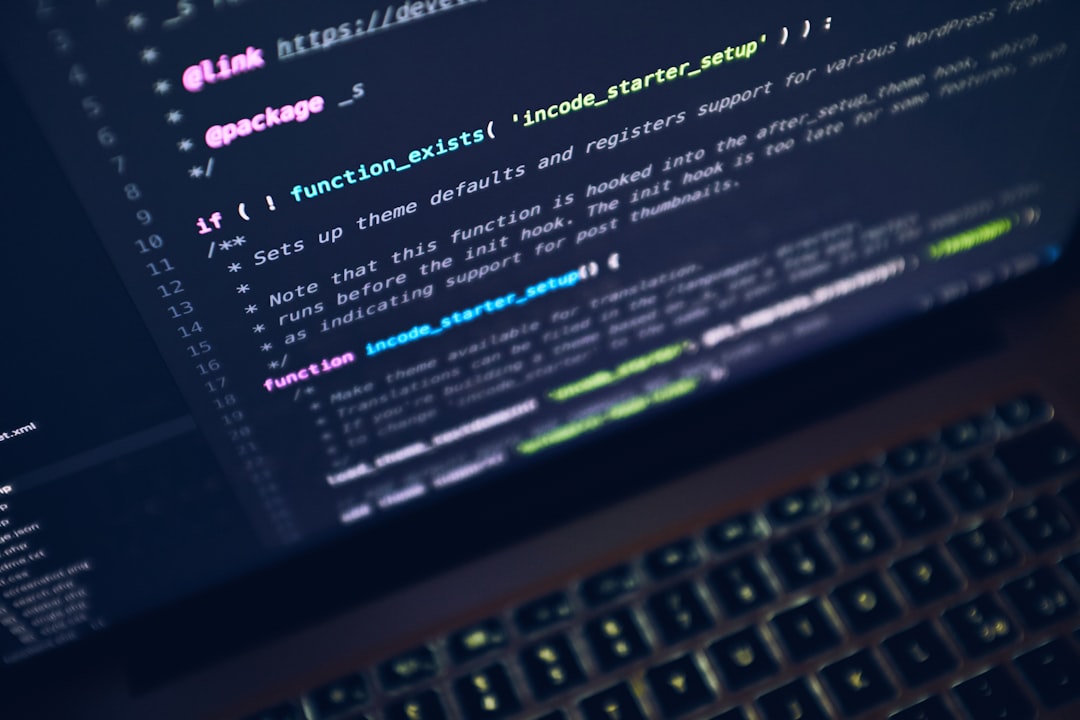
Node Authentication: Ensuring Secure Code Reviews
In the fast-evolving world of web development, Node.js has emerged as a popular platform for building scalable applications. However, with great power comes great responsibility, particularly when it comes to securing user authentication. Ensuring secure code reviews in Node authentication processes is crucial for protecting sensitive data and maintaining user trust. This article will explore best practices, current trends, and tools that can help developers achieve secure code reviews in Node.js applications.
The Importance of Secure Code Reviews
Code reviews serve as a critical checkpoint in the software development lifecycle. They involve a systematic examination of code to identify vulnerabilities, improve code quality, and ensure adherence to best practices. When it comes to authentication, even a minor oversight can lead to serious security breaches.
Common Vulnerabilities in Node Authentication
- Insecure Password Storage: Storing passwords in plain text or using weak hashing algorithms can expose user credentials.
- Inadequate Input Validation: Failing to sanitize user input can lead to SQL injection or other types of attacks.
- Token Mismanagement: Improper handling of session tokens can allow attackers to hijack user sessions.
- Lack of Rate Limiting: Without rate limiting, applications are susceptible to brute force attacks.
By performing thorough code reviews, developers can identify these vulnerabilities before the code is deployed.
Best Practices for Secure Code Reviews in Node Authentication
1. Implement Strong Password Policies
Encourage the use of complex passwords and implement measures like password strength validation during the registration process. Use libraries such as bcrypt
to hash passwords securely.
npm install bcrypt
2. Utilize Authentication Frameworks
Frameworks like Passport.js provide a robust way to handle user authentication in Node.js. They come with built-in strategies for various authentication mechanisms, reducing the likelihood of common mistakes.
3. Enforce Input Validation
Use libraries like express-validator
to validate and sanitize user inputs before processing them. This prevents malicious data from entering your application.
npm install express-validator
4. Use JSON Web Tokens (JWT) Securely
JWTs are widely used for authentication in modern web applications. Ensure that they are signed with a strong secret and that you validate them on every request. Consider using libraries like jsonwebtoken
.
npm install jsonwebtoken
5. Regular Code Reviews and Pair Programming
Encouraging regular code reviews and pair programming can significantly enhance code quality. These practices allow developers to share knowledge and catch potential issues early.
Emerging Trends in Node Authentication
1. Multi-Factor Authentication (MFA)
MFA is becoming increasingly popular as it adds an extra layer of security. This method requires users to provide two or more verification factors to gain access. Implementing MFA can be seamlessly integrated into Node.js applications using libraries like speakeasy
.
2. OAuth and OpenID Connect
These protocols are gaining traction for providing secure delegated access. They allow third-party applications to authenticate users without exposing their credentials. Utilizing libraries like passport-oauth
can simplify implementation.
Tools for Secure Code Reviews
- ESLint: This tool helps identify potential security issues in JavaScript code.
- SonarQube: A powerful tool for continuous inspection of code quality, which can detect vulnerabilities in real-time.
- Snyk: This tool allows developers to find and fix vulnerabilities in their dependencies.
Case Study: Successful Implementation of Secure Code Reviews
A leading e-commerce platform faced challenges with user authentication vulnerabilities. By implementing a structured code review process and adopting best practices, the development team could identify and rectify issues related to password management and input validation. The result was a significant reduction in security incidents, enhancing user trust and satisfaction.
Glossary of Terms
- JWT (JSON Web Token): A compact, URL-safe means of representing claims to be transferred between two parties.
- MFA (Multi-Factor Authentication): A security mechanism in which the user is required to present two or more separate forms of identification.
- OAuth: An open standard for access delegation, commonly used as a way to grant websites or applications limited access to user information.
Further Reading and Resources
- Node.js Authentication with Passport.js
- Secure Password Storage in Node.js
- Understanding JSON Web Tokens
By adhering to secure code review practices in Node authentication, developers can significantly enhance the security posture of their applications. As you continue to improve your skills, consider subscribing to relevant newsletters, sharing this article, or trying out the recommended tools mentioned above. Together, we can create a more secure web environment.