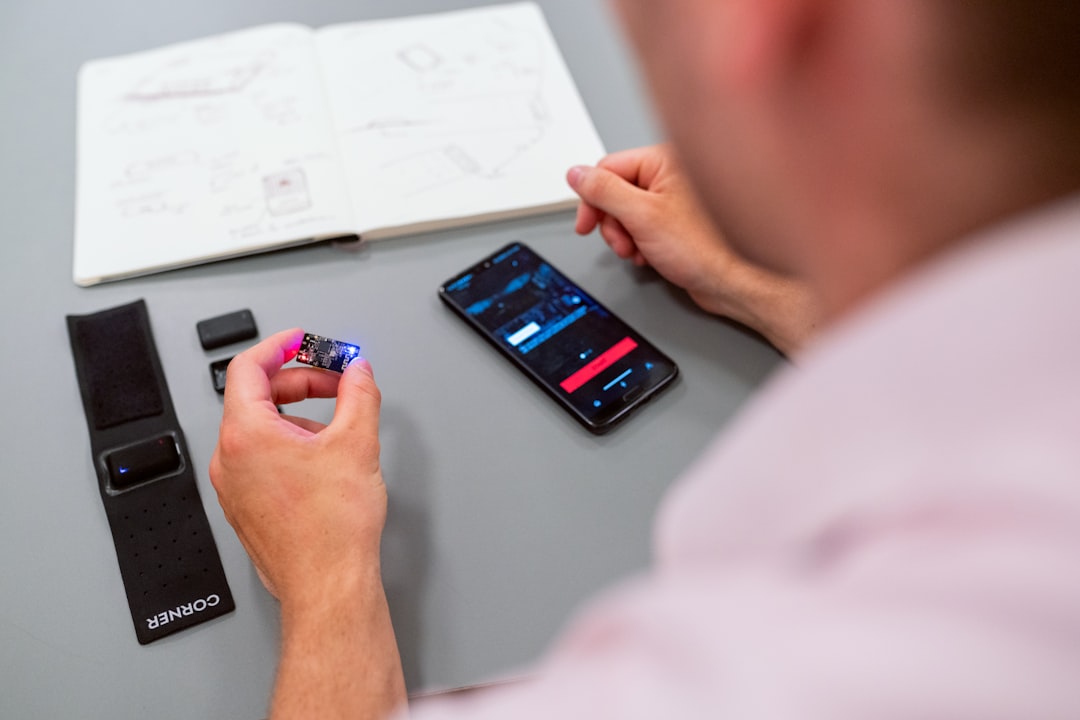
Clever Coding Techniques to Boost Your Programming Skills
Programming is an ever-evolving field, and mastering it requires an arsenal of clever coding techniques. These techniques not only enhance your coding skills but also improve your productivity and problem-solving abilities. In this article, we’ll explore various clever coding techniques that can help you become a more proficient programmer.
Understanding Code Readability
One of the most essential techniques in programming is writing readable code. Code readability allows others (and your future self) to understand your logic quickly. Here are some tips to enhance code readability:
Use Descriptive Variable Names
Instead of using generic names like x
or temp
, opt for descriptive names that convey the purpose of the variable. For instance, use userAge
instead of age
.
Consistent Indentation and Formatting
Maintaining a consistent style in your indentation and formatting is crucial. Tools like Prettier or ESLint can help automate this process, ensuring uniformity throughout your codebase.
Embrace Modular Programming
Modular programming involves breaking down your code into smaller, reusable components. This technique enhances maintainability and facilitates debugging. Here’s how to implement modular programming:
Functions and Classes
Utilize functions and classes to encapsulate specific functionalities. For example, in Python, you can define a function to calculate the area of a rectangle:
def calculate_area(length, width):
return length * width
Code Libraries
Leverage existing libraries and frameworks to avoid reinventing the wheel. For instance, using React for front-end development can save you time and effort on building user interfaces.
Utilize Version Control Systems
Version control systems (VCS) like Git are indispensable in today’s collaborative programming environment. They help you track changes, collaborate with others, and revert to previous versions if necessary.
Branching Strategies
Adopt a branching strategy such as Git Flow to manage your features and releases effectively. This approach allows you to develop new features in isolation without disrupting the main codebase.
Commit Messages
Write clear and descriptive commit messages to provide context for your changes. A well-written commit message can be invaluable for future reference.
Implement Automated Testing
Automated testing is a clever technique that ensures your code works as intended. By writing tests, you can catch bugs early in the development process.
Unit Testing
Unit tests verify the smallest parts of your code. For example, using the unittest
framework in Python, you can test the calculate_area
function:
import unittest
class TestAreaCalculation(unittest.TestCase):
def test_area(self):
self.assertEqual(calculate_area(5, 3), 15)
if __name__ == '__main__':
unittest.main()
Integration Testing
Integration tests check how different modules work together, ensuring that changes in one part of the code do not negatively impact others.
Leverage Code Reviews
Participating in code reviews is an excellent way to learn from your peers and gain new perspectives on coding practices. Providing constructive feedback can also help you solidify your understanding of various techniques.
Peer Programming
Engaging in peer programming sessions can foster knowledge sharing and collaboration. This technique allows you to learn new tricks and best practices directly from fellow developers.
Stay Updated with Emerging Trends
The technology landscape is constantly changing, and staying updated with emerging trends is crucial. Follow influential blogs, attend webinars, and participate in coding boot camps to enhance your skills.
Resources to Explore
Conclusion
Boosting your programming skills requires a combination of clever coding techniques, continuous learning, and collaboration. By focusing on code readability, modular programming, version control, automated testing, and code reviews, you can significantly enhance your abilities as a programmer.
For more advanced techniques and insights, consider exploring additional resources or subscribing to newsletters from reputable coding platforms. Sharing this article can also help others in their programming journey.
Further Reading
- Understanding Modularity in Programming
- The Importance of Code Reviews
- Introduction to Automated Testing
Glossary of Terms
- Version Control System (VCS): A tool that helps manage changes to source code over time.
- Modular Programming: A software design technique that emphasizes separating functionality into independent, interchangeable modules.
- Automated Testing: The use of software to execute tests on software applications.
By applying these clever coding techniques, you can take your programming skills to new heights and stay ahead in the fast-paced tech landscape. Happy coding!